I would like to share this informative article which explains programming in simple and effective way. This is part of an IEEE article.
Some people feel that “getting” object-oriented programming is a difficult,time-consuming process. But does it need to be that hard? And is the difficulty even specific to OO programming? Many of the cornerstones of OO programming benefit other programming paradigms as well. Even if you’re writing shell scripts or batch files, you can use these techniques to great advantage.
What’s good code?
There are many aspects to writing good code, but most of these hinge on a single underlying quality: flexibility. Flexibility means that you can change the code easily, adapt it to new and revised circumstances, and use it in contexts other than those originally intended.
Why does code need to be flexible? It’s largely because of us humans. We misunderstand communications (be they written or oral). Requirements change. We build the right thing the wrong way, or if we manage to build something the right way, it turns out to be the wrong thing by the time we’re done.
Despite our fondest wishes, we’ll never get it right the first time. Our mistakes lie in continually assuming that we can and in searching for salvation in new programming languages, better processes, or new IDEs. In-stead, we need to realize that software must be soft: it has to be easy to change because it will change despite our misguided efforts otherwise.
Capers Jones, in his book Software Assessments, Benchmarks, and Best Practices (Addi-son-Wesley, 2000), showed that requirements change at a rate of about 2 percent per month(which really starts to add up after a year or two). But the problem with changes to projects is by no means limited to the common scape-goat of “requirements,” nor is it limited to the software industry.
According to a study of building construction in the UK (“Rethinking Construction,”Construction Task Force report to the Deputy Prime Minister, 1998), some 30 percent of rework isn’t due to requirements changes at all. It’s due to mistakes: plain, old human errors, such as cutting a joist 2 inches too short. Using the wrong kind of nail. Cutting the window in the wrong wall. It’s just human nature that we’ll get some things wrong,so what differentiates software quality is how well—and how quickly we can fix or change something. Flexible code can be changed easily and cheaply, regardless of whether the change is necessitated by volatile requirements or our own misunderstanding.
Most of the important lessons to be learned about object technology—how to avoid many common mistakes and keep code flexible—can be summed up in one sentence: “Keep it DRY, keep it shy, and tell the other guy.” Let’s take a look at what that means and how you can apply these lessons to all good code, not just OO code.
Keep it DRY
Our DRY (Don’t Repeat Yourself)principle deals with knowledge representation in programs (see The Pragmatic Programmer, Addison-Wesley,2000). It’s a powerful idea that states:
Every piece of knowledge must have a single, unambiguous, and authoritative representation within a system.
In other words, you should represent any idea, any scrap of knowledge,in a system in just one place. You might end up with physical copies of code for various reasons (middle ware and data-base products could impose this restriction, for instance). But only one of these physical representations is the authoritative source. Ideally, you’d be able to automatically generate or pro-duce the non authoritative sources from the single authoritative source.
Why go to all this trouble? So that when a code change is required, you only have to make it in one place. Any-thing else is a recipe for disaster, introducing inconsistencies and potentially hard-to-find bugs.
DRY applies to code but also to every other part of the system and to developers’ daily lives—build processes,documentation, database schema, code reviews, and so on.
Keep it shy
The best code is very shy. Like a four-year old hiding behind a mother’s skirt, code shouldn’t reveal too much of itself and shouldn’t be too nosy into others affairs.
But you might find that your shy code grows up too fast, shedding its demure shyness in favor of wild promiscuity. When code isn’t shy, you’ll get unwanted coupling; these axes of ill-advised coup-ling include static, dynamic, domain, and temporal.
Static coupling exists when a piece of code requires another piece of code to compile. This isn’t a bad or evil thing far from it. Even the canonical“Hello World” program requires the standard I/O library and such. But you have to be aware of accidentally dragging in more than you need.
Inheritance is infamous for dragging in a lot of excess baggage. Often it’s more efficient, more flexible, and safer to use delegation instead of inheritance(which should be reserved for true is a relationships, not has-a or uses-a). Shy people don’t talk to strangers, and shy code should be equally wary of other code that wants to come along for the ride.
Dynamic coupling occurs when apiece of code uses another piece of code at runtime. This can get seriously out offhand using a style we call the “train wreck” .
To get the state for an order, this code has to have detailed knowledge of an address, a customer, and an order—and rely on these three components’ implied hierarchal structure. If any-thing in that mix changes, we’re introuble; this code will break. Shy code only talks to code it deals with directlyand doesn’t daisy-chain through tostrangers as in the previous example.
Domain coupling takes place whenbusiness rules and policies become em-bedded in code. Again, that’s not neces-sarily a bad thing unless mirroring real-world changes becomes difficult. If thereal world is particularly volatile, putthe business rules in metadata, either ina database or property file. Keep thecode shy by not being too nosy aboutthe details: the code can act as an en-gine for the business rules. The rulescan change at whim, and the code willmerrily process them without anychange to the code itself. Small inter-preters work well for this (reallysmall—like a case statement, not a largeyacc/lex endeavor).
Temporal coupling appears whenyou have a dependency on time—eitheron things that must occur in a certainorder, at a certain time, by a certaintime, or worse, at the same time. Al-ways plan on writing concurrent codebecause the odds are good that it willend up that way anyhow, and you’ll geta better design as a fringe benefit. Yourcode shouldn’t care about what elsemight be happening at the same time; itshould just work regardless.
Code shouldn’t be nosy. I used tohave a neighbor who would peerhawklike over her kitchen sink out thefront window and keep track of everyneighbor’s comings and goings. Her lifehung at the mercy of every whim of theentire neighborhood; it wasn’t ahealthy position for her to be in, and itisn’t a healthy position for your codeeither. A big part of not being nosy liesin our next item.
Tell the other guy
One of our favorite OO principles is“Tell, Don’t Ask” (see IEEE Software,Jan./Feb. 2003, p. 10).
To recap briefly: as an industry, we’vecome to think of software in terms offunction calls. Even in OO systems, we view an object’s behavioral interface as aset of function calls. That’s really not ahelpful metaphor. Instead of calling soft-ware a function, view it as sending amessage.
“Sending a message” to an objectconveys an air of apathy. I’ve just sentyou an order (or a request), and I don’treally care who or what you are or (es-pecially) how you do it. Just get itdone. This service-oriented, operation-centric viewpoint is critical to goodcode. Apathy toward the details, in thiscase, is just the right approach. You tellan object what to do; you don’t ask itfor data (too many details) and attemptto do the work yourself.
By “telling the other guy” in thisway, you ensure an imperative codingstyle that keeps your code from be-coming too nosy and from getting in-volved in details that it shouldn’t careabout. Such involvement would makeyour code much more vulnerable tochange. To make this work in a sys-tem, you’ll need to preserve the com-monsense semantics of commands(that is, every object that has a printmethod should behave similarly whencalled).
This isn’t an OO-specific tech-nique either. Even shell scripts canbenefit from this approach. In fact, acommon Linux command employspolymorphism at the command line.The command fsck (which is not acartoon swear word—really) per-forms a file system check. When youinvoke fsck, it determines the file sys-tem type and then runs a delegate,such as fsck.ext2, fsck.msdos,or fsck.vfat, that performs the ac-tual tests for that kind of file system.But you, as the requester, don’t care.You tell the system to check the diskvia an fsck command and it just doesit. It’s just the right amount of apathy.
So remember to “keep it DRY, keepit shy, and tell the other guy.”
Saturday, February 24, 2007
Saturday, February 17, 2007
Is India Really Rising As They Say?
As we are calling ourself as 'Rising India', 'Growing India' and with other catchy words, Are we really in a position now to praise ourself in such a over hyped way? The positive changes must be felt in the grass roots of the country and should be widely appreciated around the world by all walks of people. But this is not in our case. Whoever saying this "Rising India" is just conducting it as another commercial movement to reap the benefits by making use of patriotism. Think, how sending SMS to some XYZ number will help make India grow better? Only those asking to send and companies providing the services will grow and definitely not India. But people who are conducting the campaign says, It's reflecting the true story. But truly one thing will happen if we take the arguments rightly, i.e. promoting positive thought or wave among us we will sure have sort of positive influence.
I recently read an article about this on Editorial oh International Herald Tribune.
Here is that article authored by Philip Bowring.
"I've been bullish on India for the past 17 years, but now I'm nervous. One does not need to be in the country to be deluged by "India rising" triumphalism. The BBC World Service is providing an endlessly repeated series on the subject. Morgan Stanley's star economist Stephen Roach has followed the crowd and returned from the subcontinent with "great enthusiasm" for the "magic of its entrepreneurial spirit." Fortune magazine advises us to look out for more multibillion-dollar acquisitions by globalizing Indian companies.
A resurgence of Indian pride is understandable after decades when India was ignored by the Western media and viewed with disdain by fast-growing East Asia. Such a boost to national self- confidence must be of long-term benefit and create a dynamic of rising expectations.
But there are too many signs of an overconfidence that looks more and more like hubris. If suddenly deflated it could undercut the basis on which Indian optimism is built — that India can compete in a globalizing world and one day equal China in economic weight.
The hype about ethnic Indian talent is a reminder that a decade ago much the same thing was being said of ethnic Chinese. Back then, the world was caught up in the "miracle" of Southeast Asian growth, fueled, it was said, by the business skills and networks of overseas Chinese. Success was attributed to the culture of Confucius, who believed in a hierarchical society directed by a wise elite. "Asian values" were equated with Confucian ones. The "global Chinese" story — while not a myth — was overblown and finally punctured by the Asian economic crisis.
There are other reasons to worry now about the India hype. It is all very well for Indians to express racial pride over the success of Mittal in gaining control of European Arcelor to become the world's biggest steelmaker. But why, it might be asked, has the Indian-born, London-dwelling Lakshmi Mittal invested so little in India itself? And where would India be if its markets were as open as those of Europe, an openness which enabled Mittal to buy Arcelor?
The Tata Group's acquisition of the Anglo-Dutch steel group Corus raises other concerns. Maybe there are synergies and Tata can acquire technology. But, again, one may ask why Tata, a 100- year-old family conglomerate, is investing so heavily outside India when India offers the greatest growth potential of any major steel market. Its current steel output of 44 million tons is one-tenth that of China.
Contrast the effort by Tata to buy into the international big league with that of Posco of South Korea. Its rise from nothing to become the world's third largest producer and a leader in steel technology was achieved through organic internal growth and investment in research — just as Japan's was a generation earlier. Although Posco was protected by the government, it was always under pressure to produce quality steel at prices that kept South Korea's shipbuilding and other steel-using industries competitive.
Indian overseas acquisitions have been possible not so much because the acquirers are especially rich or dominant in their industries, but because it has been so easy to borrow. Indian companies are the beneficiaries, for now, of the same global liquidity bubble that is producing multibillion-dollar private equity takeovers and has helped the Indian stock market rise fourfold since 2003.
Thus, Indian companies are investing more overseas than foreigners are investing in India. Of course some acquisitions are in fields where India does lead — software and generic pharmaceuticals. Some are driven by business logic. But others do more to swell Indian pride than boost the Indian economy.
At home, Indian investors have been helped by an unsustainable rate of growth in bank credit — 20 percent last year. The fact is that India remains a capital-short country. The growth of its gross domestic product has been stimulated by a rise in the investment rate from around 25 percent of GDP to 30 percent. But even more is needed to sustain growth, and even the present rate may prove hard to maintain when global conditions become tighter.
As it is, India's private-sector savings surplus has fallen sharply while the public-sector deficit remains very high. The serious deterioration now occurring in the current account will probably crimp India's growth, push interest rates back up and prick the stock bubble.
Enthusiasm about India's global role as a manufacturer, given its supply of labor and vast domestic market, is fine in theory, but it must be tempered by the reality of high tariffs and a huge manufacturing trade deficit. India is more dependent than ever on exports of services and raw materials, and on workers' remittances.
Long term, I remain bullish on India. But it is time for a reality check."
I recently read an article about this on Editorial oh International Herald Tribune.
Here is that article authored by Philip Bowring.
"I've been bullish on India for the past 17 years, but now I'm nervous. One does not need to be in the country to be deluged by "India rising" triumphalism. The BBC World Service is providing an endlessly repeated series on the subject. Morgan Stanley's star economist Stephen Roach has followed the crowd and returned from the subcontinent with "great enthusiasm" for the "magic of its entrepreneurial spirit." Fortune magazine advises us to look out for more multibillion-dollar acquisitions by globalizing Indian companies.
A resurgence of Indian pride is understandable after decades when India was ignored by the Western media and viewed with disdain by fast-growing East Asia. Such a boost to national self- confidence must be of long-term benefit and create a dynamic of rising expectations.
But there are too many signs of an overconfidence that looks more and more like hubris. If suddenly deflated it could undercut the basis on which Indian optimism is built — that India can compete in a globalizing world and one day equal China in economic weight.
The hype about ethnic Indian talent is a reminder that a decade ago much the same thing was being said of ethnic Chinese. Back then, the world was caught up in the "miracle" of Southeast Asian growth, fueled, it was said, by the business skills and networks of overseas Chinese. Success was attributed to the culture of Confucius, who believed in a hierarchical society directed by a wise elite. "Asian values" were equated with Confucian ones. The "global Chinese" story — while not a myth — was overblown and finally punctured by the Asian economic crisis.
There are other reasons to worry now about the India hype. It is all very well for Indians to express racial pride over the success of Mittal in gaining control of European Arcelor to become the world's biggest steelmaker. But why, it might be asked, has the Indian-born, London-dwelling Lakshmi Mittal invested so little in India itself? And where would India be if its markets were as open as those of Europe, an openness which enabled Mittal to buy Arcelor?
The Tata Group's acquisition of the Anglo-Dutch steel group Corus raises other concerns. Maybe there are synergies and Tata can acquire technology. But, again, one may ask why Tata, a 100- year-old family conglomerate, is investing so heavily outside India when India offers the greatest growth potential of any major steel market. Its current steel output of 44 million tons is one-tenth that of China.
Contrast the effort by Tata to buy into the international big league with that of Posco of South Korea. Its rise from nothing to become the world's third largest producer and a leader in steel technology was achieved through organic internal growth and investment in research — just as Japan's was a generation earlier. Although Posco was protected by the government, it was always under pressure to produce quality steel at prices that kept South Korea's shipbuilding and other steel-using industries competitive.
Indian overseas acquisitions have been possible not so much because the acquirers are especially rich or dominant in their industries, but because it has been so easy to borrow. Indian companies are the beneficiaries, for now, of the same global liquidity bubble that is producing multibillion-dollar private equity takeovers and has helped the Indian stock market rise fourfold since 2003.
Thus, Indian companies are investing more overseas than foreigners are investing in India. Of course some acquisitions are in fields where India does lead — software and generic pharmaceuticals. Some are driven by business logic. But others do more to swell Indian pride than boost the Indian economy.
At home, Indian investors have been helped by an unsustainable rate of growth in bank credit — 20 percent last year. The fact is that India remains a capital-short country. The growth of its gross domestic product has been stimulated by a rise in the investment rate from around 25 percent of GDP to 30 percent. But even more is needed to sustain growth, and even the present rate may prove hard to maintain when global conditions become tighter.
As it is, India's private-sector savings surplus has fallen sharply while the public-sector deficit remains very high. The serious deterioration now occurring in the current account will probably crimp India's growth, push interest rates back up and prick the stock bubble.
Enthusiasm about India's global role as a manufacturer, given its supply of labor and vast domestic market, is fine in theory, but it must be tempered by the reality of high tariffs and a huge manufacturing trade deficit. India is more dependent than ever on exports of services and raw materials, and on workers' remittances.
Long term, I remain bullish on India. But it is time for a reality check."
Friday, February 16, 2007
India's Growth Depends On 'US'
I came across this very thoughtful speech from the President of India Dr. A. P. J. Abdul Kalam......... It's very long but worth spending 15 mins.
The President of India DR. A. P. J. Abdul Kalam's Speech in Hyderabad.
'ASK WHAT WE CAN DO FOR INDIA AND DO WHAT HAS TO BE DONE TO MAKE INDIA WHAT AMERICA AND OTHER WESTERN COUNTRIES ARE TODAY'
Lets do what India needs from us.
Thank you,
Dr. Abdul Kalam
(PRESIDENT OF INDIA)
The President of India DR. A. P. J. Abdul Kalam's Speech in Hyderabad.
Why is the media here so negative? Why are we in India so embarrassed to recognize our own strengths, our achievements? We are such a great nation. We have so many amazing success stories but we refuse to acknowledge them. Why? We are the first in milk production. We are number one in Remote sensing satellites. We are the second largest producer of wheat. We are the second largest producer of rice. Look at Dr. Sudarshan, he has transferred the tribal village into a self-sustaining, self-driving unit. There are millions of such achievements but our media is only obsessed in the bad news and failures and disasters. I was in Tel Aviv once and I was reading the Israeli newspaper. It was the day after a lot of attacks and bombardments and deaths had taken place. The Hamas had struck. But the front page of the newspaper had the picture of a Jewish gentleman who in five years had transformed his desert into an orchid and a granary. It was this inspiring picture that everyone woke up to. The gory details of killings, bombardments, deaths, were inside in the newspaper, buried among other news.
In India we only read about death, sickness, terrorism, crime. Why are we so NEGATIVE? Another question: Why are we, as a nation so obsessed with foreign things? We want foreign T. Vs, we want foreign shirts. We want foreign technology.
Why this obsession with everything imported. Do we not realize that self-respect comes with self-reliance? I was in Hyderabad giving this lecture, when a 14 year old girl asked me for my autograph. I asked her what her goal in life is. She replied: I want to live in a developed India. For her, you and I will have to build this developed India. You must proclaim. India is not an under-developed nation; it is a highly developed nation. Do you have 10 minutes? Allow me to come back with a vengeance.
Got 10 minutes for your country? If yes, then read; otherwise, choice is yours.
YOU say that our government is inefficient.
YOU say that our laws are too old.
YOU say that the municipality does not pick up the garbage.
YOU say that the phones don't work, the railways are a joke,
The airline is the worst in the world, mails never reach their destination.
YOU say that our country has been fed to the dogs and is the absolute pits.
YOU say, say and say. What do YOU do about it? Take a person on his way to Singapore. Give him a name - YOURS. Give him a face - YOURS. YOU walk out of the airport and you are at your International best. In Singapore you don't throw cigarette butts on the roads or eat in the stores. YOU are as proud of their Underground links as they are. You pay $5 (approx. Rs. 60) to drive through Orchard Road (equivalent of Mahim Causeway or Pedder Road) between 5 PM and 8 PM. YOU come back to the parking lot to punch your parking ticket if you have over stayed in a restaurant or a shopping mall irrespective of your status identity... In Singapore you don't say anything, DO YOU? YOU wouldn't dare to eat in public during Ramadan, in Dubai. YOU would not dare to go out without your head covered in Jeddah. YOU would not dare to buy an employee of the telephone exchange in London at 10 pounds (Rs.650) a month to, 'see to it that my STD and ISD calls are billed to someone else.'YOU would not dare to speed beyond 55 mph (88 km/h) in Washington and then tell the traffic cop, 'Jaanta hai main kaun hoon (Do you know who I am?). I am so and so's son. Take your two bucks and get lost.' YOU wouldn't chuck an empty coconut shell anywhere other than the garbage pail on the beaches in Australia and New Zealand. Why don't YOU spit Paan on the streets of Tokyo? Why don't YOU use examination jockeys or buy fake certificates in Boston??? We are still talking of the same YOU. YOU who can respect and conform to a foreign system in other countries but cannot in your own. You who will throw papers and cigarettes on the road the moment you touch Indian ground. If you can be an involved and appreciative citizen in an alien country, why cannot you be the same here in India?
Once in an interview, the famous Ex-municipal commissioner of Bombay, Mr.Tinaikar, had a point to make. 'Rich people's dogs are walked on the streets to leave their affluent droppings all over the place,' he said. 'And then the same people turn around to criticize and blame the authorities for inefficiency and dirty pavements. What do they expect the officers to do? Go down with a broom every time their dog feels the pressure in his bowels? In America every dog owner has to clean up after his pet has done the job. Same in Japan. Will l the Indian citizen do that here?' He's right. We go to the polls to choose a government and after that forfeit all responsibility. We sit back wanting to be pampered and expect the government to do everything for us whilst our contribution is totally negative. We expect the government to clean up but we are not going to stop chucking garbage all over the place nor are we going to stop to pick a up a stray piece of paper and throw it in the bin. We expect the railways to provide clean bathrooms but we are not going to learn the proper use of bathrooms. We want Indian Airlines and Air India to provide the best of food and toiletries but we are not going to stop pilfering at the least opportunity. This applies even to the staff who is known not to pass on the service to the public. When it comes to burning social issues like those related to women, dowry, girl child! and others, we make loud drawing room protestations and continue to do the reverse at home. Our excuse? 'It's the whole system which has to change, how will it matter if I alone forego my sons' rights to a dowry.' So who's going to change the system? What does a system consist of ? Very conveniently for us it consists of our neighbors, other households, other cities, other communities and the government. But definitely not me and YOU. When it comes to us actually making a positive contribution to the system we lock ourselves along with our families into a safe cocoon and look into the distance at countries far away and wait for a Mr.Clean to come along & work miracles for us with a majestic sweep of his hand or we leave the country and run away. Like lazy cowards hounded by our fears we run to America to bask in their glory and praise their system. When New York becomes insecure we run to England. When England experiences unemployment, we take the next flight out to the Gulf. When the Gulf is war struck, we demand to be rescued and brought home by the Indian government. Everybody is out to abuse and rape the country. Nobody thinks of feeding the system. Our conscience is mortgaged to money.
Dear Indians, The article is highly thought inductive, calls for a great deal of introspection and pricks one's conscience too.... I am echoing J.F. Kennedy's words to his fellow Americans to relate to Indians.....
In India we only read about death, sickness, terrorism, crime. Why are we so NEGATIVE? Another question: Why are we, as a nation so obsessed with foreign things? We want foreign T. Vs, we want foreign shirts. We want foreign technology.
Why this obsession with everything imported. Do we not realize that self-respect comes with self-reliance? I was in Hyderabad giving this lecture, when a 14 year old girl asked me for my autograph. I asked her what her goal in life is. She replied: I want to live in a developed India. For her, you and I will have to build this developed India. You must proclaim. India is not an under-developed nation; it is a highly developed nation. Do you have 10 minutes? Allow me to come back with a vengeance.
Got 10 minutes for your country? If yes, then read; otherwise, choice is yours.
YOU say that our government is inefficient.
YOU say that our laws are too old.
YOU say that the municipality does not pick up the garbage.
YOU say that the phones don't work, the railways are a joke,
The airline is the worst in the world, mails never reach their destination.
YOU say that our country has been fed to the dogs and is the absolute pits.
YOU say, say and say. What do YOU do about it? Take a person on his way to Singapore. Give him a name - YOURS. Give him a face - YOURS. YOU walk out of the airport and you are at your International best. In Singapore you don't throw cigarette butts on the roads or eat in the stores. YOU are as proud of their Underground links as they are. You pay $5 (approx. Rs. 60) to drive through Orchard Road (equivalent of Mahim Causeway or Pedder Road) between 5 PM and 8 PM. YOU come back to the parking lot to punch your parking ticket if you have over stayed in a restaurant or a shopping mall irrespective of your status identity... In Singapore you don't say anything, DO YOU? YOU wouldn't dare to eat in public during Ramadan, in Dubai. YOU would not dare to go out without your head covered in Jeddah. YOU would not dare to buy an employee of the telephone exchange in London at 10 pounds (Rs.650) a month to, 'see to it that my STD and ISD calls are billed to someone else.'YOU would not dare to speed beyond 55 mph (88 km/h) in Washington and then tell the traffic cop, 'Jaanta hai main kaun hoon (Do you know who I am?). I am so and so's son. Take your two bucks and get lost.' YOU wouldn't chuck an empty coconut shell anywhere other than the garbage pail on the beaches in Australia and New Zealand. Why don't YOU spit Paan on the streets of Tokyo? Why don't YOU use examination jockeys or buy fake certificates in Boston??? We are still talking of the same YOU. YOU who can respect and conform to a foreign system in other countries but cannot in your own. You who will throw papers and cigarettes on the road the moment you touch Indian ground. If you can be an involved and appreciative citizen in an alien country, why cannot you be the same here in India?
Once in an interview, the famous Ex-municipal commissioner of Bombay, Mr.Tinaikar, had a point to make. 'Rich people's dogs are walked on the streets to leave their affluent droppings all over the place,' he said. 'And then the same people turn around to criticize and blame the authorities for inefficiency and dirty pavements. What do they expect the officers to do? Go down with a broom every time their dog feels the pressure in his bowels? In America every dog owner has to clean up after his pet has done the job. Same in Japan. Will l the Indian citizen do that here?' He's right. We go to the polls to choose a government and after that forfeit all responsibility. We sit back wanting to be pampered and expect the government to do everything for us whilst our contribution is totally negative. We expect the government to clean up but we are not going to stop chucking garbage all over the place nor are we going to stop to pick a up a stray piece of paper and throw it in the bin. We expect the railways to provide clean bathrooms but we are not going to learn the proper use of bathrooms. We want Indian Airlines and Air India to provide the best of food and toiletries but we are not going to stop pilfering at the least opportunity. This applies even to the staff who is known not to pass on the service to the public. When it comes to burning social issues like those related to women, dowry, girl child! and others, we make loud drawing room protestations and continue to do the reverse at home. Our excuse? 'It's the whole system which has to change, how will it matter if I alone forego my sons' rights to a dowry.' So who's going to change the system? What does a system consist of ? Very conveniently for us it consists of our neighbors, other households, other cities, other communities and the government. But definitely not me and YOU. When it comes to us actually making a positive contribution to the system we lock ourselves along with our families into a safe cocoon and look into the distance at countries far away and wait for a Mr.Clean to come along & work miracles for us with a majestic sweep of his hand or we leave the country and run away. Like lazy cowards hounded by our fears we run to America to bask in their glory and praise their system. When New York becomes insecure we run to England. When England experiences unemployment, we take the next flight out to the Gulf. When the Gulf is war struck, we demand to be rescued and brought home by the Indian government. Everybody is out to abuse and rape the country. Nobody thinks of feeding the system. Our conscience is mortgaged to money.
Dear Indians, The article is highly thought inductive, calls for a great deal of introspection and pricks one's conscience too.... I am echoing J.F. Kennedy's words to his fellow Americans to relate to Indians.....
'ASK WHAT WE CAN DO FOR INDIA AND DO WHAT HAS TO BE DONE TO MAKE INDIA WHAT AMERICA AND OTHER WESTERN COUNTRIES ARE TODAY'
Lets do what India needs from us.
Thank you,
Dr. Abdul Kalam
(PRESIDENT OF INDIA)
Metcalfe's law
While browsing on net, I found out law that particular has some osrt of usage in our day to day activities and applications or services one provide.
Here is the description of the law. Interpret it and try to think in terms of your view, then you will understand the usage.
Metcalfe's law states that the value of a telecommunications network is proportional to the square of the number of users of the system (n2). First formulated by Robert Metcalfe in regard to Ethernet, Metcalfe's law explains many of the network effects of communication technologies and networks such as the Internet and World Wide Web.
The law has often been illustrated using the example of fax machines: A single fax machine is useless, but the value of every fax machine increases with the total number of fax machines in the network, because the total number of people with whom each user may send and receive documents increases.
Revisions
There have been subsequent revisions, however, to the estimated value added; for example, that Metcalfe's law may exaggerate that benefit. Since a user cannot connect to itself, the reasoning goes, the actual calculation is the number of diagonals and sides in an n-gon.
n(n-1)
------
2
Andrew Odlyzko and Benjamin Tilly published a preliminary paper which concluded Metcalfe's law significantly overestimates the value of adding connections. The rule of thumb becomes: "the value of a network with n members is not n squared, but rather n times the logarithm of n." Their primary justification for this is the idea that not all potential connections in a network are equally valuable. For example, most people call their families a great deal more often than they call strangers in other countries, and so do not derive the full value n from the phone service. In the July 2006 IEEE Spectrum, Bob Briscoe, Odlyzko and Tilly state more succinctly: "Metcalfe's Law is Wrong". Robert Metcalfe responded to the IEEE article, defending his namesake law, on a partner's blog.
In contrast, Reed's law asserts that Metcalfe's law understates the value of adding connections. Not only is a member connected to the entire network as a whole, but also to many significant subsets of the whole. These subsets add value independent of either the individual or the network as a whole. Including subsets into the calculation of the value of the network increases the value faster than just including individual nodes.
Applications of Metcalfe's law
Metcalfe's Law can be applied to more than just telecommunications devices. Metcalfe's Law can be applied to almost any computer systems that exchange data. Examples of devices include:
* Telephones
* FAXs
* Operating systems
* Applications
* Social networking websites
Metcalfe's Law frequently predicts whether a single vendor or interface standard will tend to dominate a marketplace. This has implications for whether an innovative solution can enter a marketplace that requires different interfaces.
The same law can be applied to other technological systems, such as personal genome sequencing. As more human genomes are sequenced and tied to personal health information in an interconnected system, the value of the information that a personal genome can contribute to personal health grows.
Thanks to Wikipedia for providing this information.
Thursday, February 08, 2007
Wednesday, February 07, 2007
Good Standards To Follow in PHP
In Software Development, most of the programmers have faced a very familiar situation of understanding other people codes for revamping or optimization. It's really challenging task to work in a maintenance project that demands cracking another programmers logic and thoughts. I equally love working in maintenance/optimization projects as like the new development projects. But as programmer I always strive hard to follow common standard while coding for a project. Coding style can vary by a bit for different project to avoid disinterest. Coding standards can be followed to maintain a consistent programming style throughout the core code base. If programmers follow guidelines it will be easier for others to understand and to maintain what has been developed if the project needs to optimized even after their stint.
I got sometime to work on finalizing standardizing the code styles that needs to be followed for future development... As these are very generic and can be useful for others I'm glad to share those.
Sections:
Class Names
Method Names
Private class members
Example
Class Attribute Names
Method Argument Names
Variable Names
Array Element
Global Variables
No Magic Numbers
Comments at top of file:
Usage of ?:
I got sometime to work on finalizing standardizing the code styles that needs to be followed for future development... As these are very generic and can be useful for others I'm glad to share those.
Sections:
- Naming Convention
- Indentation/Tabs/Space Policy
- Usage of Parens () with Key Words and Functions Policy
- Control Structures
- Function Calls
- Function Definitions
- Alignment of Declaration Blocks
- No Magic Numbers
- Comments
- Others
- Usage of ?:
- Do Not Default If Test to Non-Zero
- PHP Code Tags
- Miscellaneous
Class Names
- Use upper case letters as word separators, lower case for the rest of a word
- First character in a name is upper case
- No underbars ('_')
- When confronted with a situation where you could use an all upper case abbreviation instead use an initial upper case letter followed by all lower case letters. No matter what
class NameOneTwo
class Name
class GetHtmlStatistic
Method Names
- Use the same rules as class
Connect()
GetData()
BuildSomeWidget()
Private class members
- Class members that are intended to be used only from within the same class in which they are declared are preceded by a single underscore.
private $_mStatusProtected class members
_Sort()
_InitTree()
- Class members that are intended to be used only from within the same class in which they are declared or from subclasses that extend it are not preceded by a single underscore.
Example
protected $mSomevar
protected function InitTree()
Class Attribute Names
- Class member attribute names should be prefixed with the character 'm'
- After the 'm' use the same rules as for class names
- 'm' always precedes other name modifiers like 's' for static
$mVarAbc
$mErrorNumber
$msVolume
Method Argument Names
- Methods argument names should be prefixed with the character 'a'
- After the 'a' use the same rules as for class names
function StartYourEngines($aSomeEngine, $aAnotherEngine, $aAgainOne)
Variable Names
- Use all lower case letters
- Use '_' as the word separator
$error
$an_example_for_var
Array Element
- Array element names follow the same rules as a variable.
- Use '_' as the word separator.
- Don't use '-' as the word separator
- Access an array's elements with single or double quotes
$myarr['foo_bar'] = 'Hello';
Global Variables
- Global variables should be prefixed with a 'g'
global $gLogConstants
global $gTimeOut
- Constants should always be all-uppercase, with underscores to separate words
- Prefix constant names with the uppercased name of the class they are used in
GLOBAL_CONSTANT_FOR_EXStatic Variables
- Static variables may be prefixed with 's'
static $msVolumeFunction Names
static $msVolume
- Use all lower case letters with '_' as the word delimiter
function some_function()Indentation/Tabs/Space Policy
{
//code block
}
- Indent using 4 spaces for each level
- Do not use tabs, use spaces
function function()Parens () with Key Words and Functions Policy
{
if (something bad){
if (another thing bad){
while (more input){
}
}
}
}
- Do not put parens next to keywords. Put a space between
- Do put parens next to function names
- Do not use parens in return statements when it's not necessary
if (condition){Control Structures
}
while (condition){
}
strcmp($s, $s1);
return 1;
- These include if, for, while, switch, etc
- Control statements should have one space between the control keyword and opening parenthesis, to distinguish them from function calls
- Always use curly braces even in situations where they are technically optional. Having them increases readability and decreases the likelihood of logic errors being introduced when new lines are added.
if ((condition1) || (condition2)) {Function Calls
action1;
} elseif ((condition3) && (condition4)) {
action2;
} else {
defaultaction;
}
switch (condition) {
case 1:{
action1;
break;
}
case 2:{
action2;
break;
}
default:{
defaultaction;
break;
}
}
- Functions should be called with no spaces between the function name, the opening parenthesis, and the first parameter
- Give single space between commas and each parameter, and no space between the last parameter, the closing parenthesis, and the semicolon
- There should be one space on either side of an equals sign used to assign the return value of a function to a variable
foo($bar, $baz, $quux)Function Definitions
$short = foo($bar);
$long_variable = foo($baz);
- Arguments with default values go at the end of the argument list
- Depends on where you are defining the function(Class or outside), use the appropriate naming convention
function connect($aDsn, $aPersistent = false)Alignment of Declaration Blocks
{
return true;
}
- Block of declarations should be aligned
var $mDate
var& $mrDate
var& $mrName
var $mName
$mDate = 0;
$mrDate = NULL;
$mrName = 0;
$mName = NULL;
No Magic Numbers
- A magic number is a bare-naked number used in source code. It's magic because no-one has a clue what it means including the author inside 3 months.
- Instead of magic numbers use a real name that means something. You should use define() for example.
if (22 == $foo) {Correct Usage Example
start_thread();
} else if (19 == $foo) {
refund();
} else if (16 == $foo) {
infinite_loop();
}else {
blah_blah();
}
define("TIME_OUT", "22");Comments
define("ROUND_UP", "19");
define("BREAK_DOWN", "16");
if (TIME_OUT == $foo) {
start_thread();
} else if (ROUND_UP == $foo) {
refund();
} else if (BREAK_DOWN == $foo) {
infinite_loop();
}else {
blah_blah();
}
- Consider your comments a story describing the system.
- Expect your comments to be extracted by a robot and formed into a man page.
- Class comments are one part of the story, method signature comments are another part of the story, method arguments another part, and method implementation yet another part. All these parts should weave together and inform someone else at another point of time just exactly what you did and why.
Comments at top of file:
/*Comments at top of class:
* Short description for file
*
* Long description for file (if any)...
*
* @Module ModuleName
* @package PackageName
* @author Original Author
* @author Another Author
*/
/**Comments at top of function:
* Short description of class
*
* Long description of class (if any)...
* @Module ModuleName
* @package PackageName
*/
/**Others
* Short description of function
*
* Long description of function (if any)...
*
* @param $arg1 short description about first argument
* @param $arg1 short description about second argument
*
* @return short description what function will return
*
* @access specify the access type
* @static true/false
*/
function ShowResults($arg1, $arg2)
{
return 1;
}
Usage of ?:
- Put the condition in parens so as to set it off from other code
- If possible, the actions for the test should be simple functions
- Put the action for the then and else statement on a separate line unless it can be clearly put on one line
(condition) ? funct1() : func2();Do Not Default If Test to Non-Zero
(condition)
? long statement
: another long statement;
- Do not default the test for non-zero
if (FAIL != f())PHP Code Tags
is better than
if (f())
- Use standard approach to mark PHP codes like
<?phpMiscellaneous
?>
- Do not do any real work in an object's constructor. Inside a constructor initialize variables only and/or do only actions that can't fail
- There should be only one statement per line unless the statements are very closely related.
- Always document a null body for a for or while statement so that it is clear that the null body is intentional and not missing code
while ($dest++ = $src++)As usual comments are always welcome to discuss and make corrective measures.
; // VOID
Saturday, February 03, 2007
Part 3: Trip To Shimla
After a brief visit to The Ridge and The Malls road shopping, the first day in Shimla went off fine with heavy dinner at a Punjabi Restaurant in The Mall road.
Next and final day in Shimla was completely scheduled by the Himachal Pradesh Tourism's tour package. We got into their bus in morning 9.45Am. They took us first to Fagu Point, which is one of the highest peak. We spend some time there and from there we went to check out Golf Course Club. We roamed around there for a hour. All the places are covered by thick greenery. Really refreshing to see. Then from there we went to Kufri Point. Actually all the vehicles have to stop some 7 KM's before the point. There is not way vehicles can reach to that steep point. One has to reach either by walk or by riding in Horse. Me and my friend decided to go by walk just to get the feeling of Trekking and as a physical exercise. It took us One hour to reach the Point. It was not a green point actually, but filled with different activities. From Kufri Point you can see the Great China Border, Patiala Palace where the famous Shimla Agreement was signed between India and Pakistan. Also in that Point you can see a small temple called "Himalayan Temple". It was good experience. By this time evening came and we are forced to get back into Bus and returned to our starting point. As a final visit, Me and my Friend decided to visit "Jakoo Temple" which is located at very steep place. Really I should say, I climbed the "road" to reach that place. I wondered how vehicles are moving in that steep roads.
Jakoo Temple is the place where some parts of famous movie "Roja" was filmed. Oops beware of monkeys if you visit there. There is no need for any bandits to rob you, monkeys will do that instead.
Here are the pictures,
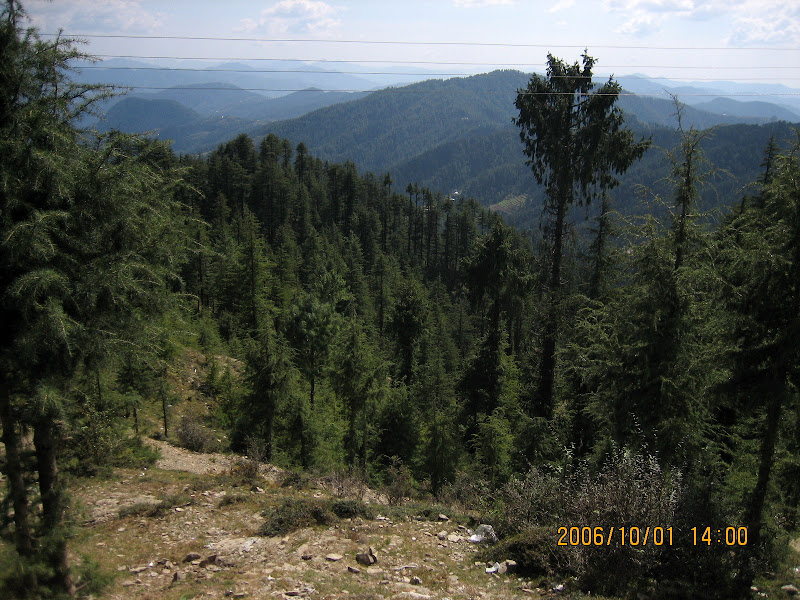
Me over an Ox in Kufri Point
The same but with some effect to symbolize the arrival of "Yema Tharamraj"
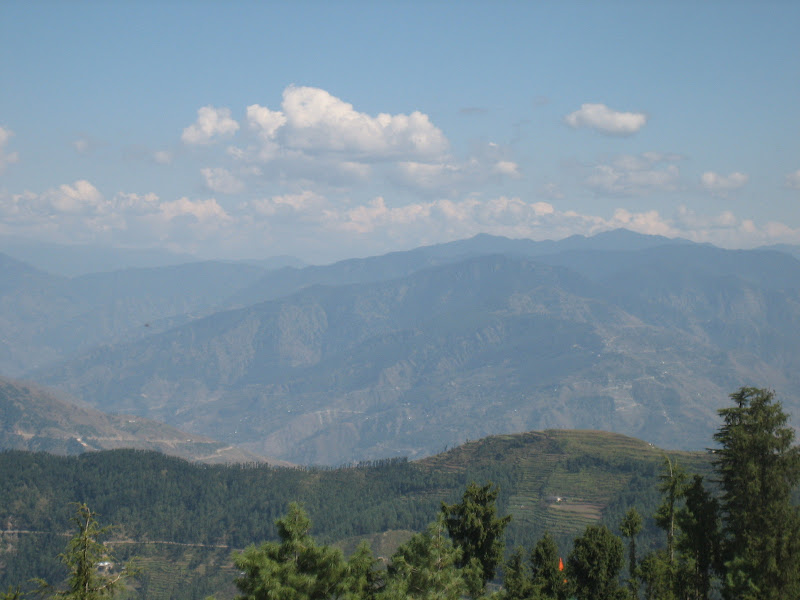
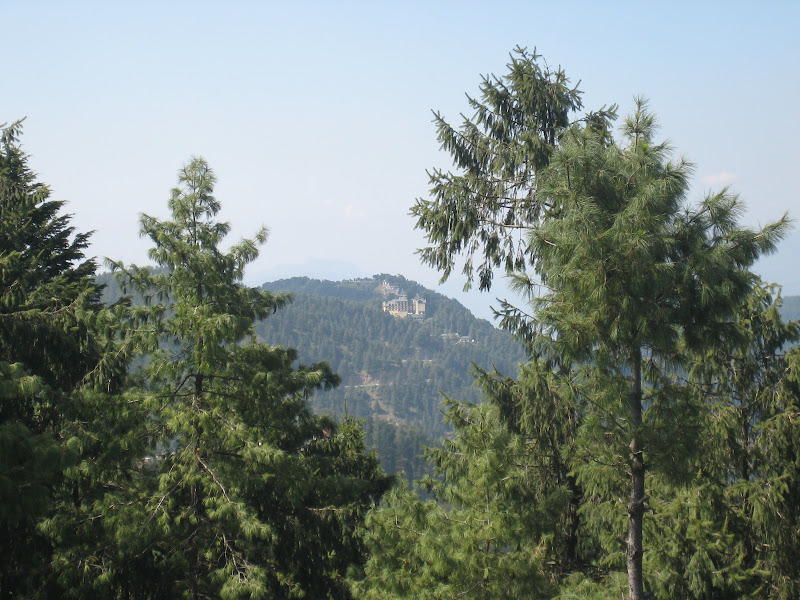
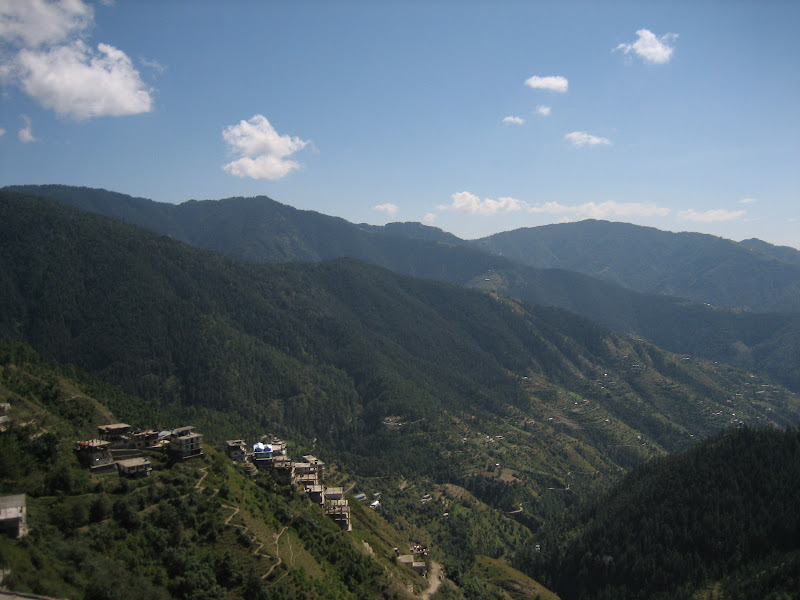
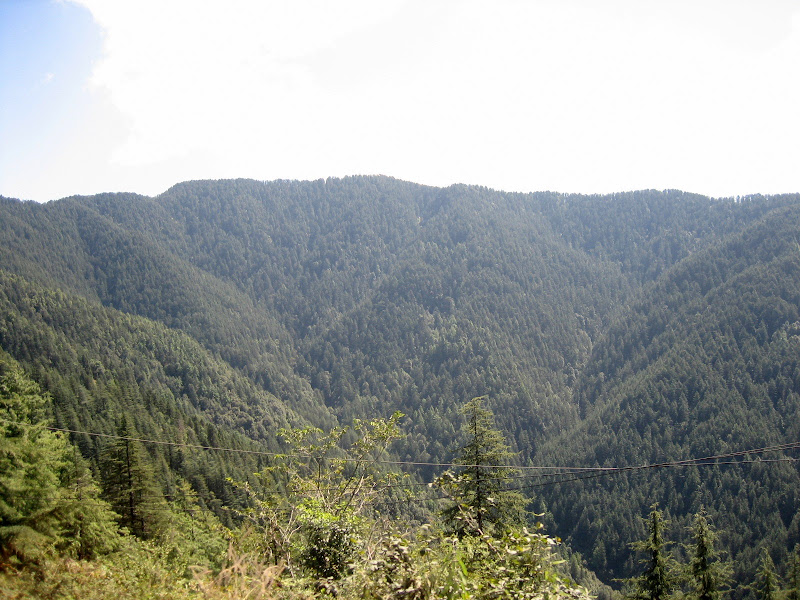
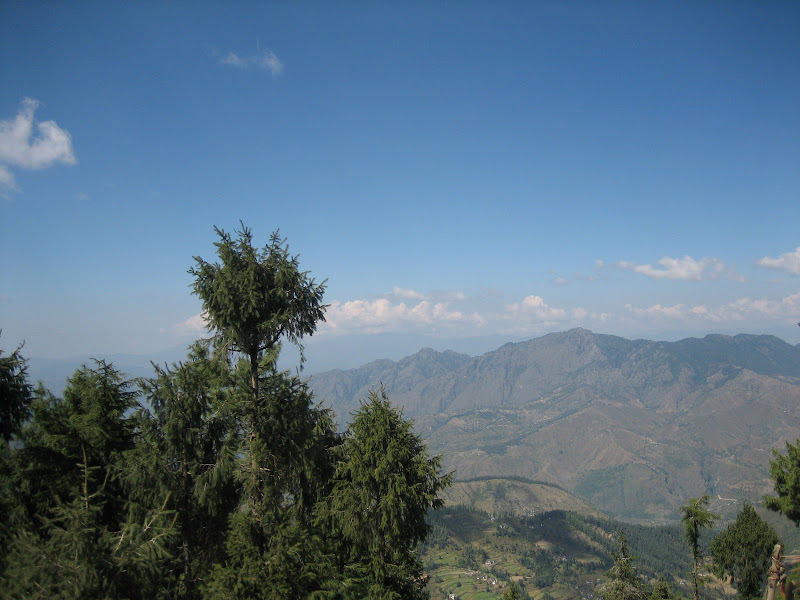
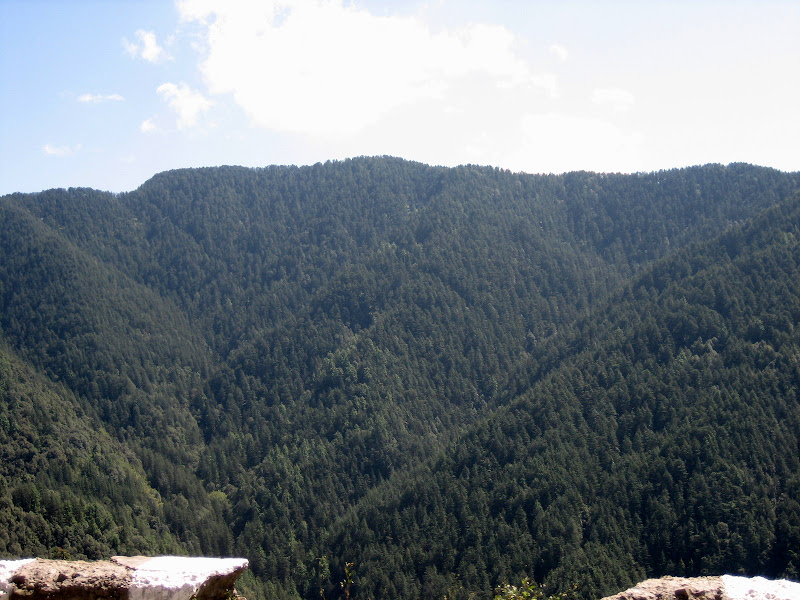
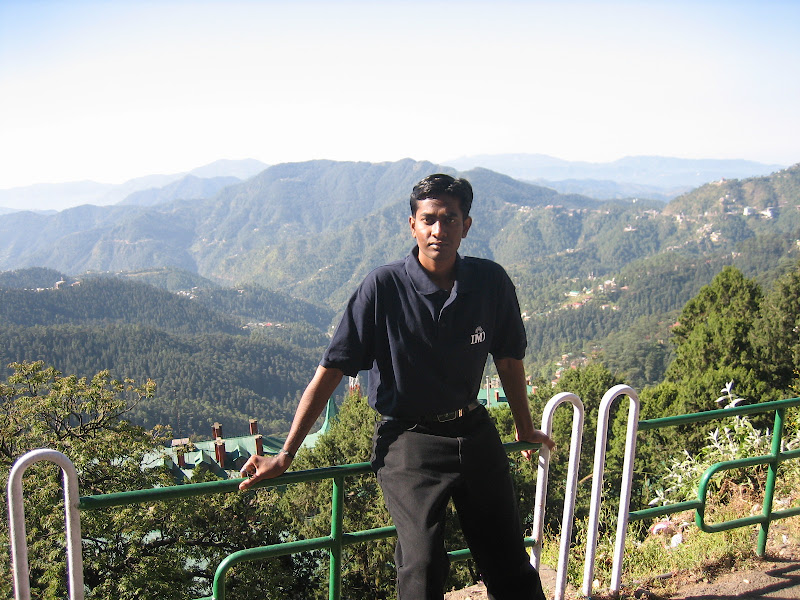
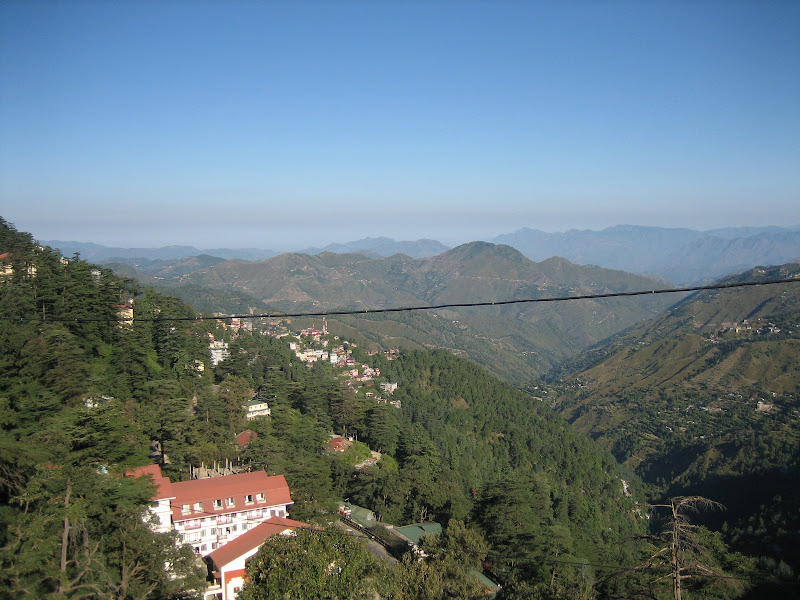
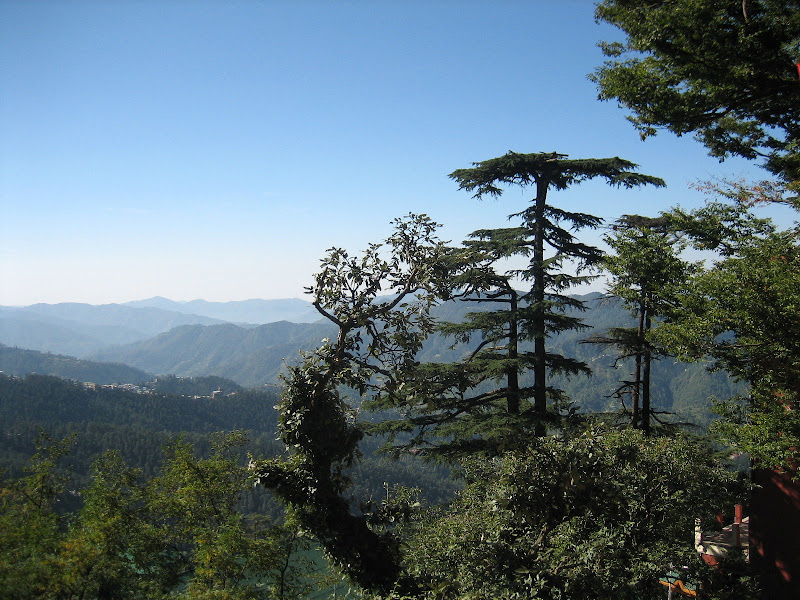
Next and final day in Shimla was completely scheduled by the Himachal Pradesh Tourism's tour package. We got into their bus in morning 9.45Am. They took us first to Fagu Point, which is one of the highest peak. We spend some time there and from there we went to check out Golf Course Club. We roamed around there for a hour. All the places are covered by thick greenery. Really refreshing to see. Then from there we went to Kufri Point. Actually all the vehicles have to stop some 7 KM's before the point. There is not way vehicles can reach to that steep point. One has to reach either by walk or by riding in Horse. Me and my friend decided to go by walk just to get the feeling of Trekking and as a physical exercise. It took us One hour to reach the Point. It was not a green point actually, but filled with different activities. From Kufri Point you can see the Great China Border, Patiala Palace where the famous Shimla Agreement was signed between India and Pakistan. Also in that Point you can see a small temple called "Himalayan Temple". It was good experience. By this time evening came and we are forced to get back into Bus and returned to our starting point. As a final visit, Me and my Friend decided to visit "Jakoo Temple" which is located at very steep place. Really I should say, I climbed the "road" to reach that place. I wondered how vehicles are moving in that steep roads.
Jakoo Temple is the place where some parts of famous movie "Roja" was filmed. Oops beware of monkeys if you visit there. There is no need for any bandits to rob you, monkeys will do that instead.
Here are the pictures,
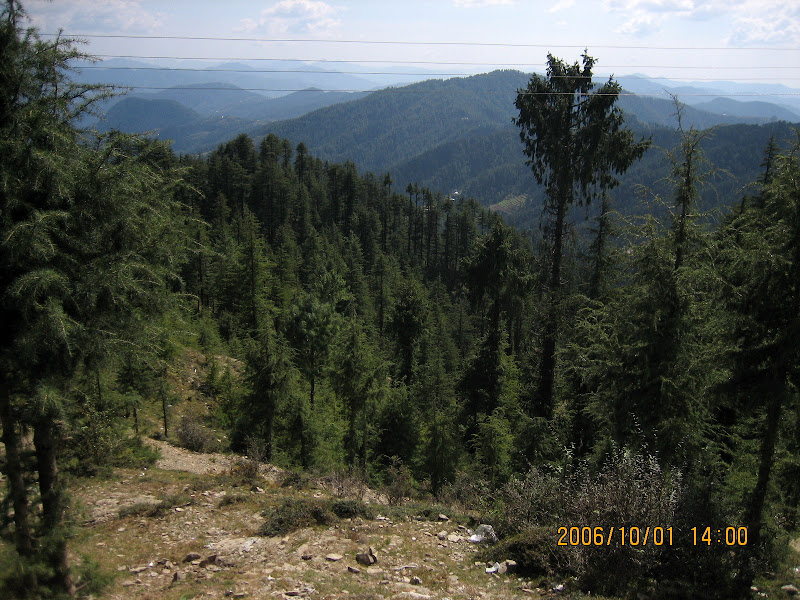
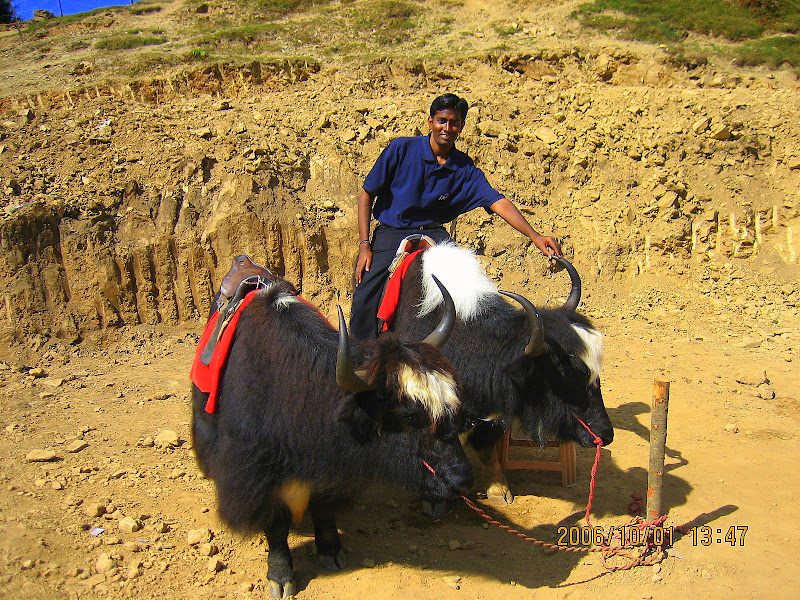
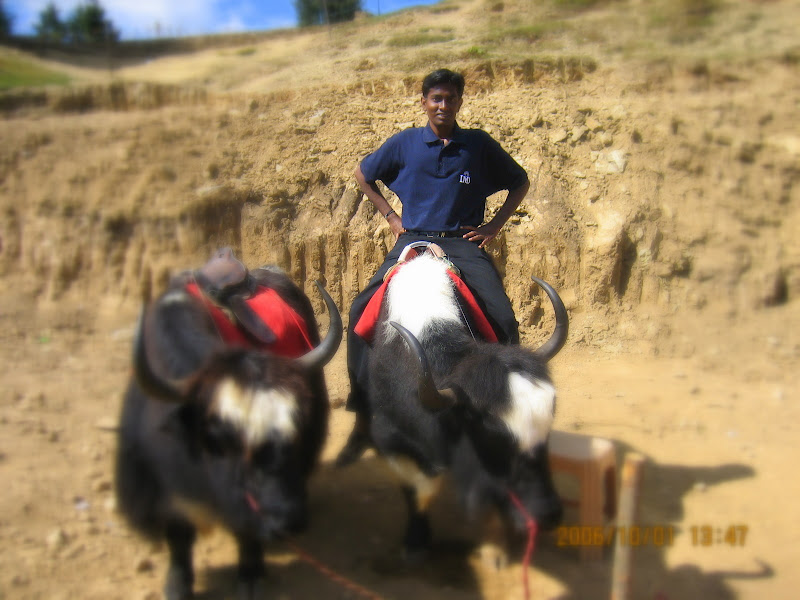
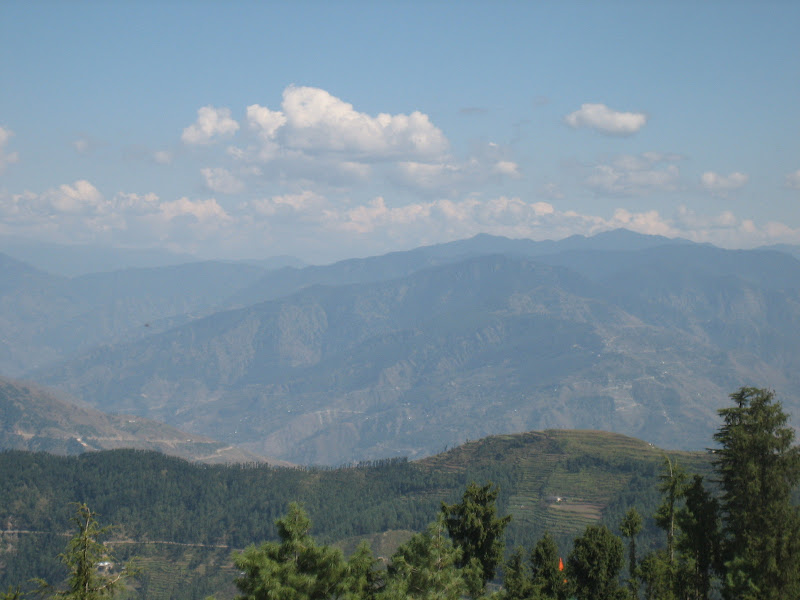
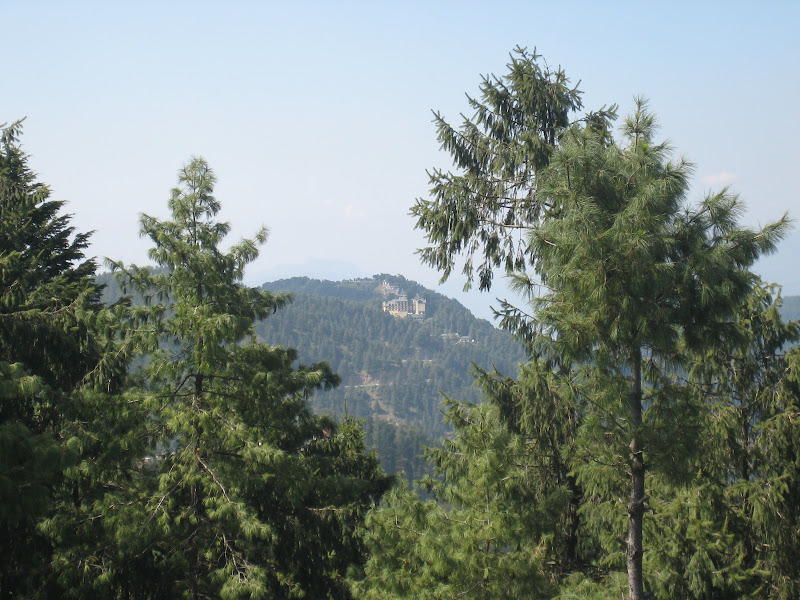
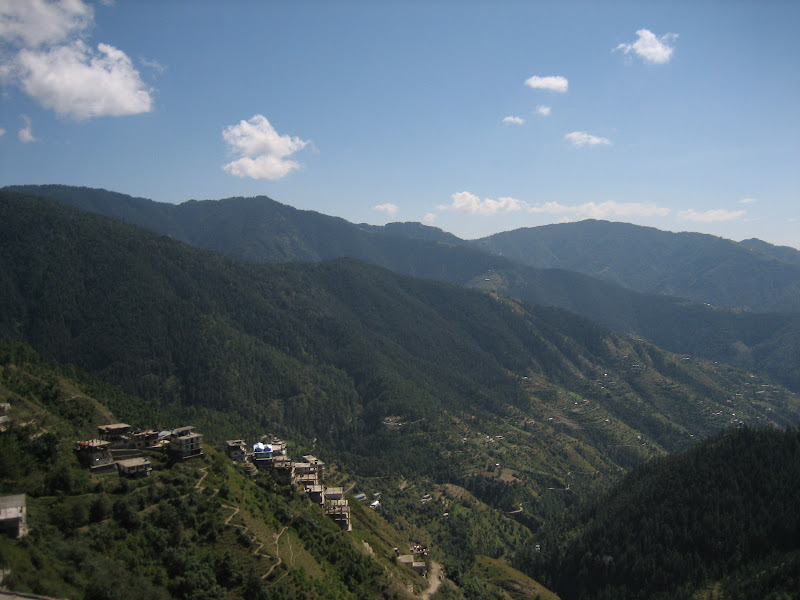
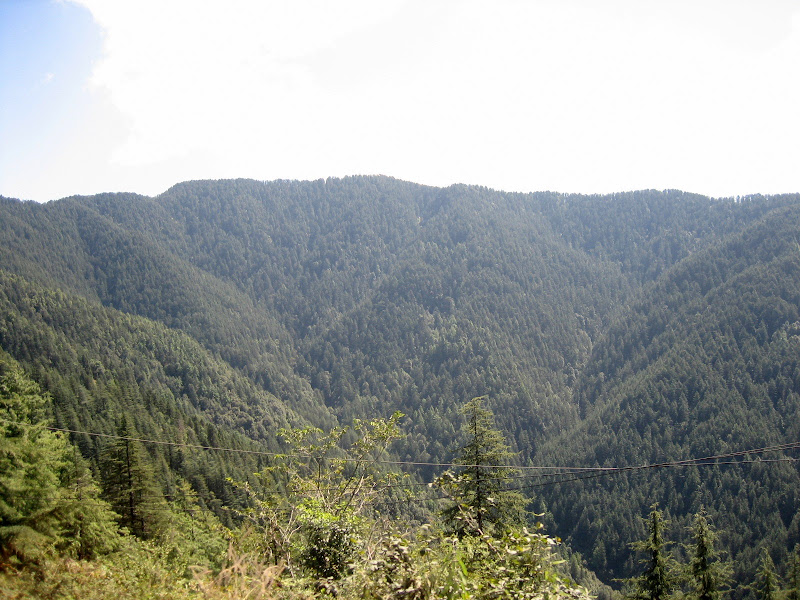
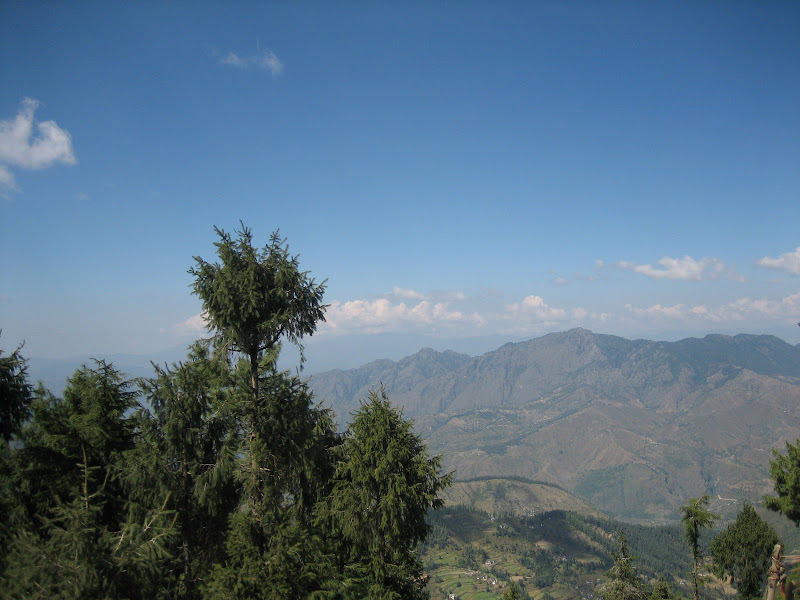
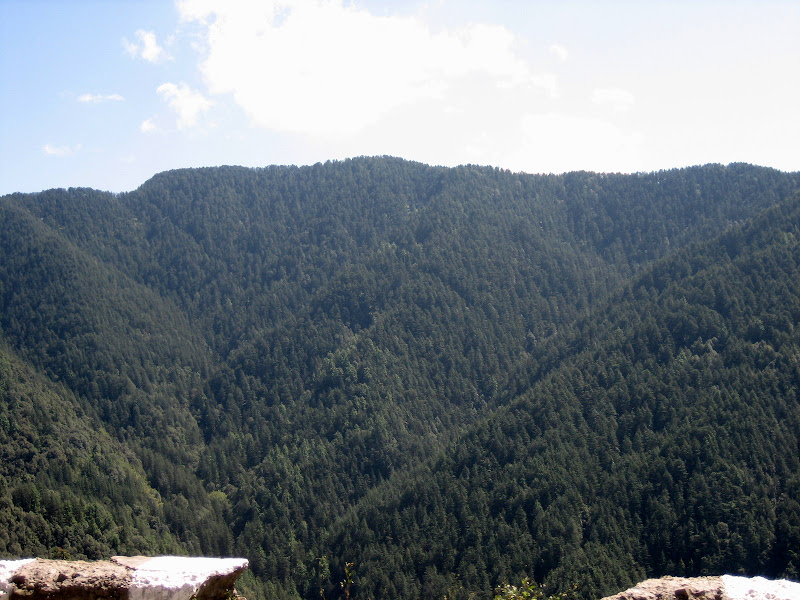
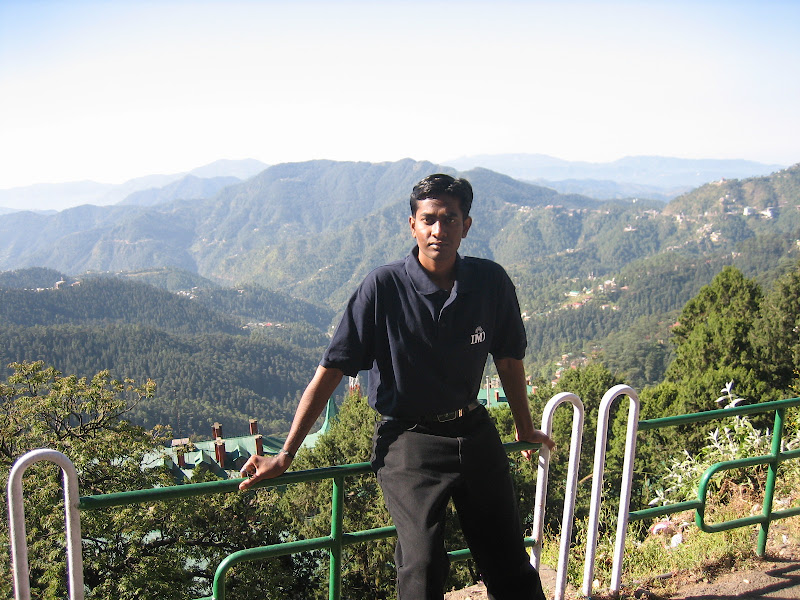
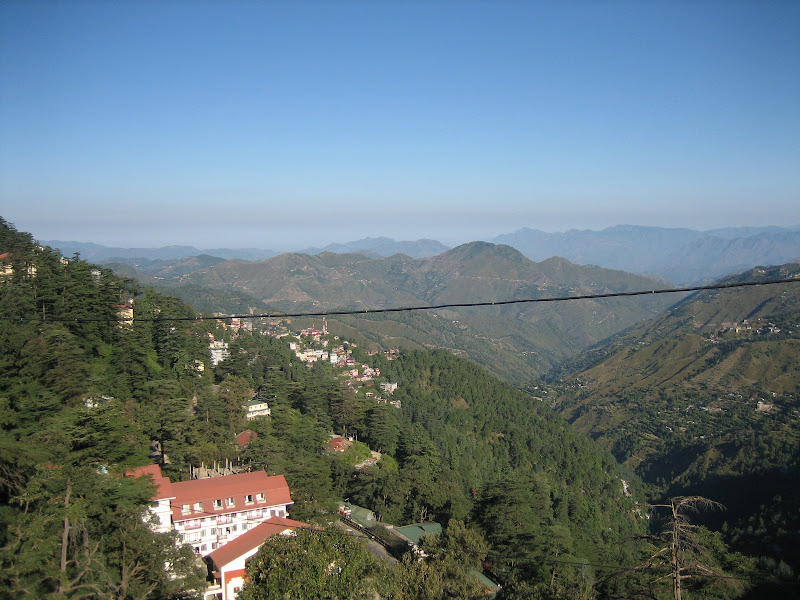
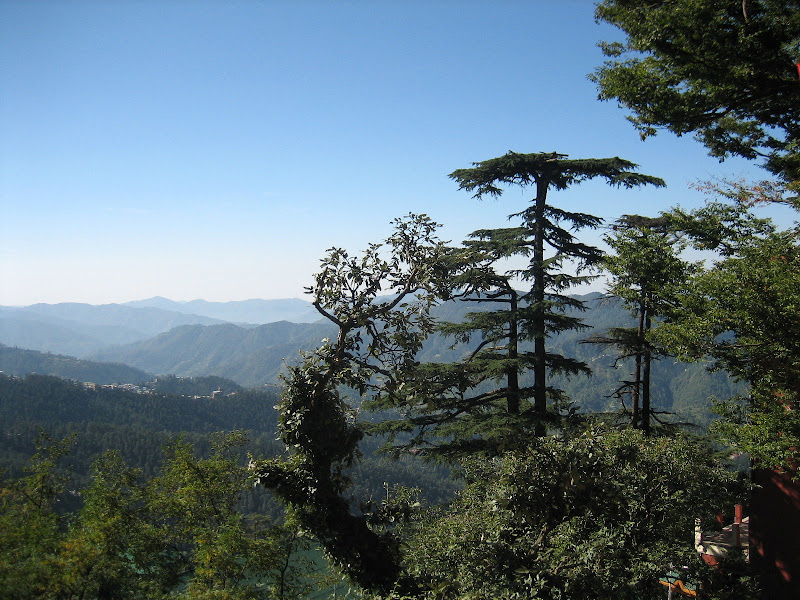
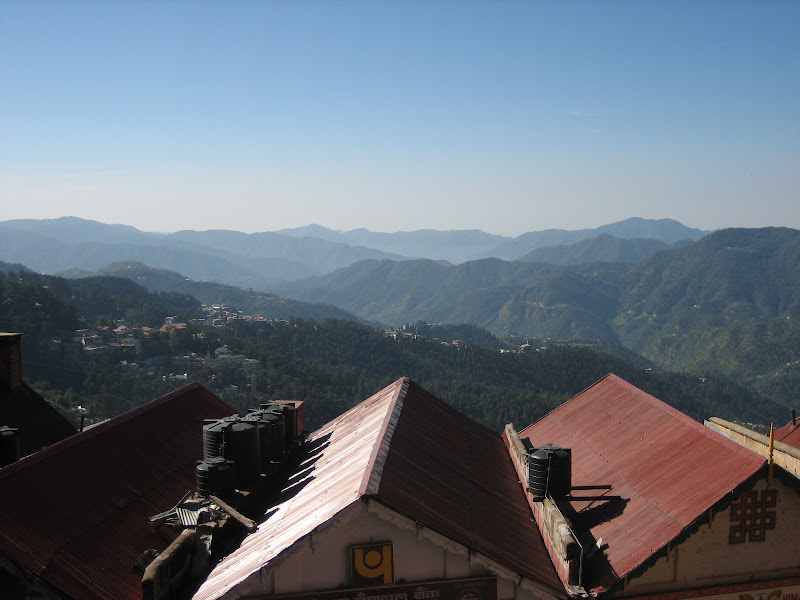
Part 2: Trip To Shimla
After a wonderful brief stay at Chandigarh, We got bus to Shimla at 9AM. Actually we planned to goto Shimla by the Toy Train. But unfortunately we didn't get ticket and it's running with jam packed crowd. We should have booked it very early, early means some 3 months advance because all the year there large amount of tourists are moving in and out of Shimla. So we need to have paucca plan to have hassle free travel. Okay but even the bus ride in the mountain ride was very interesting. We got into the bus which has a Singh as driver. He was really great driver. He seemed not et al bothered about whether take driving through the congested Kalka roads or lots of hair pin bend mountain roads. He just drive like a King riding Horse. Enjoyed his style of driving and not necessarily to say the beautiful scenes all along the road to Shimla.
It took nearly 3 hours to reach Shimla. Once we got down at Shimla, at least some 10 to 15 people came towards us, initially I thought they were auto walla or tourist agent. But later on only I found out that, they will just give directions to people for some amount. Good type of business. After getting information about the Himachel Pradesh tourist office and lodging places, we paid 20rs for his service. Then we started our novel journey to get the glimse of Queen of Himlayas. We got the first hand experience of mountain terrain. Had to walk through steep roads to goto different places. Then finally we reached our lodge which is just behind the popular place The Ridge. Here you can see statues of famous leaders of India lined up both the side of road.
Here are the pictures,
It took nearly 3 hours to reach Shimla. Once we got down at Shimla, at least some 10 to 15 people came towards us, initially I thought they were auto walla or tourist agent. But later on only I found out that, they will just give directions to people for some amount. Good type of business. After getting information about the Himachel Pradesh tourist office and lodging places, we paid 20rs for his service. Then we started our novel journey to get the glimse of Queen of Himlayas. We got the first hand experience of mountain terrain. Had to walk through steep roads to goto different places. Then finally we reached our lodge which is just behind the popular place The Ridge. Here you can see statues of famous leaders of India lined up both the side of road.
Here are the pictures,
Trip to Shimla
Here it comes finally... Yes, the long awaiting pictures of my Shimla trip. I went to Shimla with my friend Arun in the month of October,06. It was a very refreshing and wonderful trip. Also it gave me a first hand knowledge of North India. That was my first trip in North India and travelled through Kurushektra, Ambala, Chandigarh and Kalka.
I got into train at 10Pm at Nizammudin Railway Station, South Delhi. Next day morning we got down in Chandigarh Railway Station at 7AM. From the moment I stepped out of that station I felt, the city Chandigarh has lots in offer to me. From the Chandigarh station I travelled to bus station to get the bus to Shimla. As I expected, It was really a beatifull and wonderful city. Straight roads, more importantly roads were very clean. Really enjoyed the short travel in the city. By the time we reached bus station we are too hungry and wanted to refresh. So from bus station we went to near by Sector 17 market by walk. You will definitely say WoW once you get into that market. It was huge, clean and tidy market. Those old age look buildings lined up at both sides with big space between them added more beauty. After a some search, we found
a age old restaurant. Felt that I was moving back into Gandhian era. That restaurant was named after Gandhi and people worked were all wearing the Nehru style dresses which typically has white Kurda and a type of cap. Luckily they had South Indian items and more surprising fact is they menu have only South Indian items. We had hot and tasty Dosa and Idly with different North Indian cooking flavor.
Here are the pictures of my first stop over on the way to Shimla
I got into train at 10Pm at Nizammudin Railway Station, South Delhi. Next day morning we got down in Chandigarh Railway Station at 7AM. From the moment I stepped out of that station I felt, the city Chandigarh has lots in offer to me. From the Chandigarh station I travelled to bus station to get the bus to Shimla. As I expected, It was really a beatifull and wonderful city. Straight roads, more importantly roads were very clean. Really enjoyed the short travel in the city. By the time we reached bus station we are too hungry and wanted to refresh. So from bus station we went to near by Sector 17 market by walk. You will definitely say WoW once you get into that market. It was huge, clean and tidy market. Those old age look buildings lined up at both sides with big space between them added more beauty. After a some search, we found
a age old restaurant. Felt that I was moving back into Gandhian era. That restaurant was named after Gandhi and people worked were all wearing the Nehru style dresses which typically has white Kurda and a type of cap. Luckily they had South Indian items and more surprising fact is they menu have only South Indian items. We had hot and tasty Dosa and Idly with different North Indian cooking flavor.
Here are the pictures of my first stop over on the way to Shimla
Subscribe to:
Posts (Atom)